Exploring Next.js 13: getStaticProps, getStaticPaths, and getServerSideProps
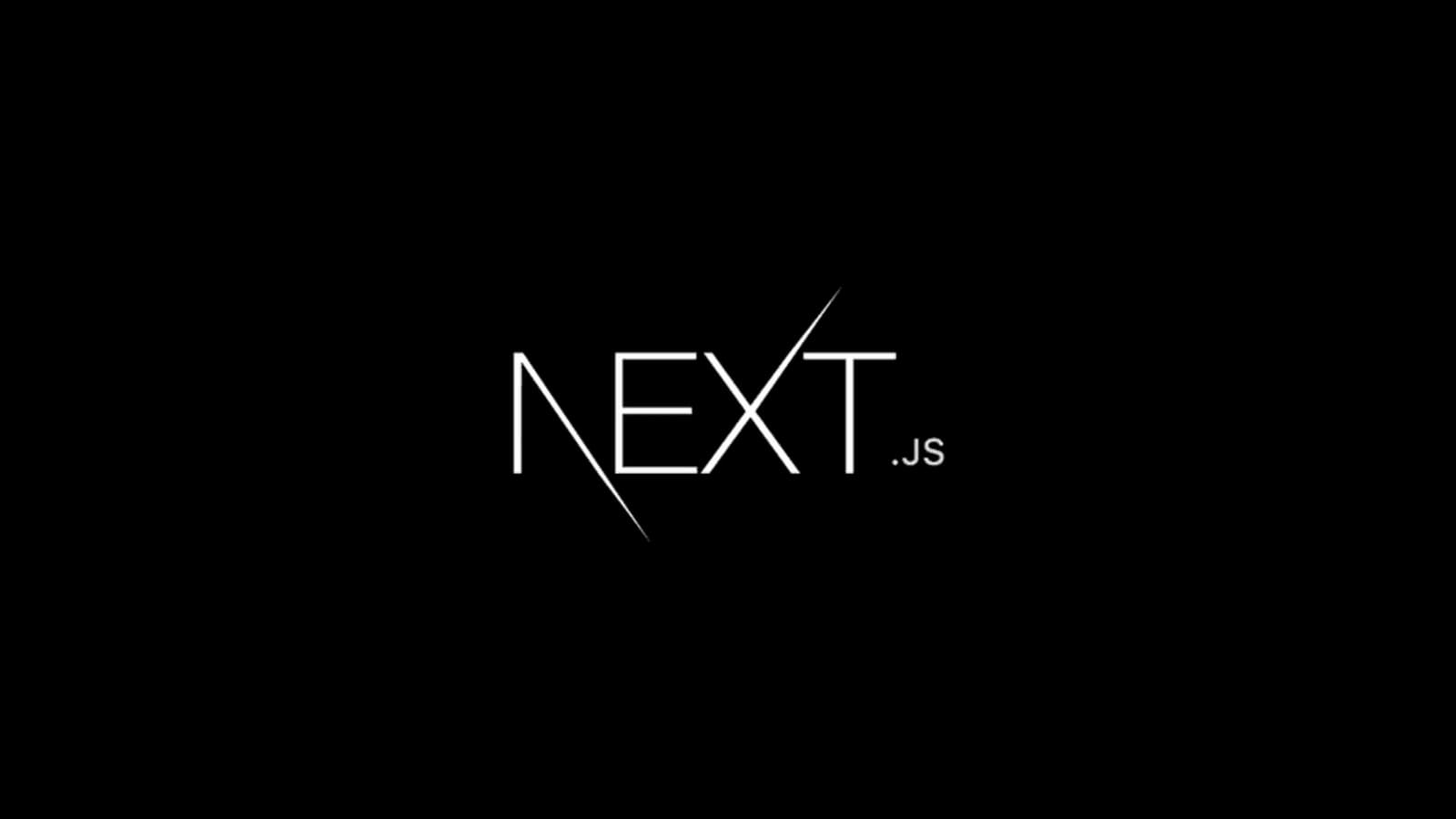
Exploring Next.js 13: getStaticProps, getStaticPaths, and getServerSideProps
- Optimize Pre-rendered Pages (getStaticProps): One of the key features introduced in Next.js 9, getStaticProps, allows developers to fetch data during the build process and pre-render pages at build time. By using this function, you can improve your application's performance and reduce the time it takes to load pages. getStaticProps works by fetching data from a data source, such as an API or a database, and returning it as props to a page component. This data is then pre-rendered into HTML at build time, ensuring that the pages served to users already contain the necessary data.
Furthermore, getStaticProps enables Next.js to automatically optimize your application by generating static HTML files for each page. These files are then cached by Next.js and served to subsequent users, eliminating the need to re-render the page for every request.
// pages/blog.ts
interface BlogProps {
posts: Post[];
}
interface Post {
id: number;
title: string;
content: string;
}
const Blog: React.FC<BlogProps> = ({ posts }) => {
// Render the blog posts
return (
<div>
{posts.map((post) => (
<div key={post.id}>
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
))}
</div>
)
}
export async function getStaticProps() {
// Fetch data from an API or database
const response = await fetch('https://api.example.com/posts')
const posts = await response.json()
return {
props: {
posts,
},
}
}
export default Blog
- Dynamic Routes Made Easy (getStaticPaths): Next.js also provides the getStaticPaths function, which is particularly useful when working with dynamic routes. With getStaticPaths, you can generate dynamic paths based on the data you fetch from an API or a database. By defining the getStaticPaths function, Next.js will pre-render all possible paths for a dynamic route at build time. This ensures that every possible variation of the dynamic route is available as a static HTML file. When a user visits one of these paths, Next.js serves the corresponding pre-rendered HTML, resulting in faster page loads and improved user experience.
// pages/blog/[slug].ts
import { GetStaticPaths, GetStaticProps } from 'next'
interface BlogPostProps {
post: Post;
}
interface Post {
id: number;
slug: string;
title: string;
content: string;
}
const BlogPost: React.FC<BlogPostProps> = ({ post }) => {
// Render the blog post
return (
<div>
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
)
}
export const getStaticPaths: GetStaticPaths = async () => {
// Fetch all blog post slugs from an API or database
const response = await fetch('https://api.example.com/posts')
const posts: Post[] = await response.json()
// Generate paths for each blog post slug
const paths = posts.map((post) => ({
params: { slug: post.slug },
}))
return {
paths,
fallback: false,
}
}
export const getStaticProps: GetStaticProps<BlogPostProps> = async ({
params,
}) => {
// Fetch data for a specific blog post based on the slug
const response = await fetch(`https://api.example.com/posts/${params.slug}`)
const post: Post = await response.json()
return {
props: {
post,
},
}
}
export default BlogPost
- On-demand Server-side Rendering (getServerSideProps): Sometimes, you may require data that cannot be pre-rendered at build time due to its dynamic nature or sensitive nature. In such cases, Next.js offers getServerSideProps, a function that allows you to fetch data on each request and pre-render the page server-side. When using getServerSideProps, the data fetching process happens on the server each time a user requests the page. This ensures that the data is always up to date and can be personalized for each user. Although server-side rendering comes with a performance cost compared to static pre-rendering, it provides flexibility when dealing with real-time or frequently updated data.
// pages/profile.ts
import { GetServerSideProps } from 'next'
interface ProfileProps {
user: User;
}
interface User {
id: number;
name: string;
email: string;
}
const Profile: React.FC<ProfileProps> = ({ user }) => {
// Render the user profile
return (
<div>
<h2>{user.name}</h2>
<p>Email: {user.email}</p>
</div>
)
}
export const getServerSideProps: GetServerSideProps<
ProfileProps
> = async () => {
// Fetch user data from an API or database
const response = await fetch('https://api.example.com/user')
const user: User = await response.json()
return {
props: {
user,
},
}
}
export default Profile
$$
$$
$$ $$