V8 Engine: the Basics
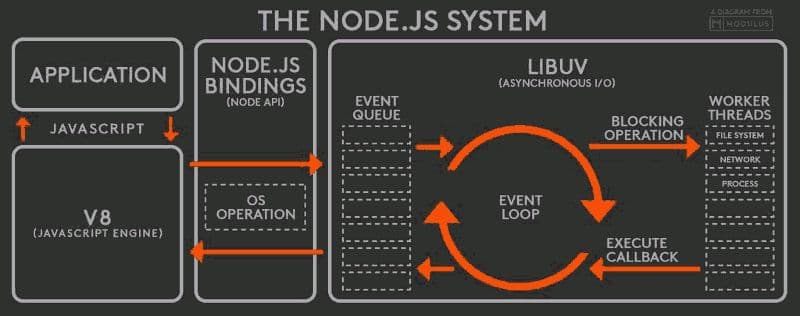
The Basics of v8 engine
The V8 engine is a high-performance JavaScript engine developed by Google for use in their Chrome browser. It was later used as the foundation for Node.js. The V8 engine compiles JavaScript code into machine code before executing it, which makes it much faster than traditional JavaScript engines that interpret code at runtime.
One of the key features of the V8 engine is its Just-In-Time (JIT) compiler. The JIT compiler compiles frequently used code into machine code at runtime, which significantly improves performance. The V8 engine also uses a garbage collector to manage memory allocation and deallocation, which reduces the risk of memory leaks.
How Node.js Uses the v8 Engine
Node.js uses the V8 engine to execute JavaScript code on the server-side. When a Node.js application starts, it creates a single thread that runs the event loop. The event loop is a mechanism that allows Node.js to handle I/O operations asynchronously, without blocking the main thread.
how-does-v8-engine-workWhen Node.js receives a request, it triggers an event that is added to the event queue. The event loop then retrieves the next event from the queue and executes it. If the event involves I/O operations, the event loop delegates the task to the underlying operating system, which handles it asynchronously. Once the I/O operation is complete, the event loop retrieves the event from the event queue and resumes execution.
The V8 engine is responsible for executing the JavaScript code associated with each event. When the V8 engine encounters a function call, it compiles the function into machine code and executes it. If the function contains a blocking I/O operation, the V8 engine hands it off to the event loop and continues executing other functions.
How it does exactly work
The Node.js v8 engine executes JavaScript code on the server-side by using a single thread that runs the event loop. When a request is received, it is added to the event queue, and the event loop retrieves the next event and executes it. The V8 engine compiles the JavaScript code associated with each event into machine code and executes it, while also delegating blocking I/O operations to the event loop. Finally, the V8 engine includes several optimizations to improve performance.
- When a Node.js application starts, it creates a single thread that runs the event loop.
- When a request is received, it is added to the event queue.
- The event loop retrieves the next event from the queue and executes it.
- If the event involves I/O operations, the event loop delegates the task to the underlying operating system, which handles it asynchronously.
- Once the I/O operation is complete, the event loop retrieves the event from the event queue and resumes execution.
- The V8 engine is responsible for executing the JavaScript code associated with each event.
- When the V8 engine encounters a function call, it compiles the function into machine code and executes it.
- If the function contains a blocking I/O operation, the V8 engine hands it off to the event loop and continues executing other functions.
- The V8 engine also includes several optimizations to improve performance, such as the use of the Cluster module, the Hidden Classes optimization, and the Crankshaft compiler.
Optimization in Node.js v8 Engine
The Node.js v8 engine includes several optimizations to improve performance. One of the most significant optimizations is the use of the Cluster module, which allows Node.js to utilize multiple processor cores. The Cluster module creates a separate process for each processor core, which enables Node.js to handle more requests simultaneously.
The v8 engine also includes a feature called the "Hidden Classes" optimization. This optimization reduces the time it takes to access object properties by creating hidden classes for objects that share the same structure. This optimization can significantly improve the performance of applications that create many objects with similar structures.
Another optimization in the v8 engine is the use of the "Crankshaft" compiler. The Crankshaft compiler optimizes frequently used code by generating optimized machine code at runtime. This optimization can significantly improve the performance of applications that have hotspots in their code.