Coolest things of modern Javascript
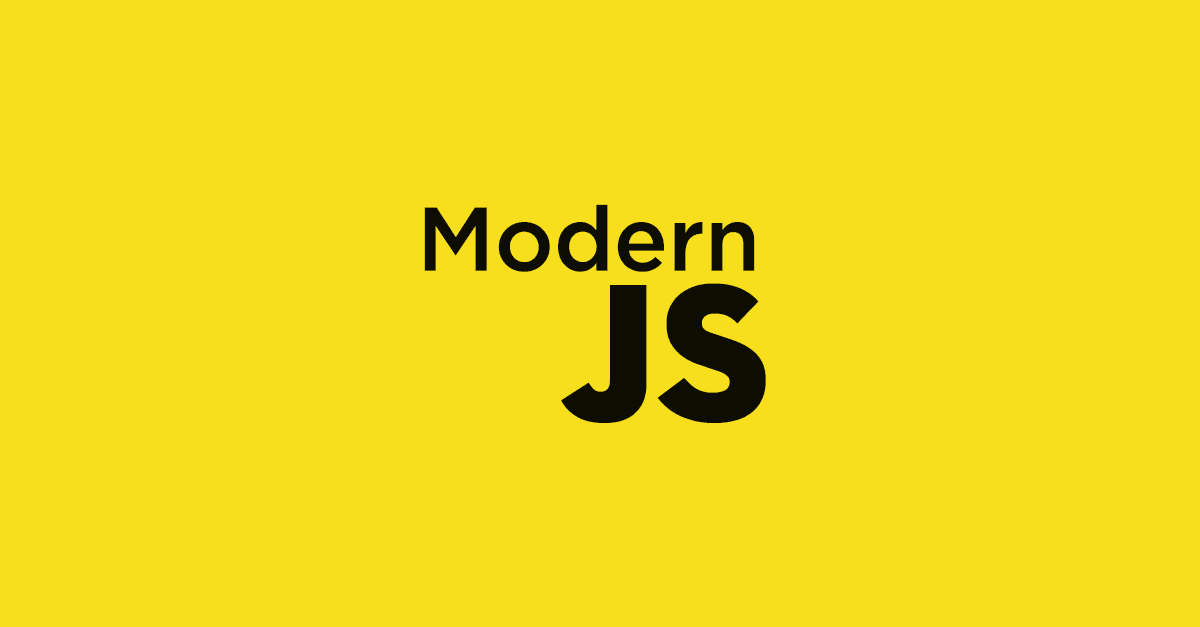
JavaScript is a dynamic, high-level programming language that is widely used for creating web applications. Over the years, JavaScript has evolved significantly, and with the release of new versions of the language, developers have access to many powerful features that can help them create better and more efficient applications. Here are some of the coolest features of modern JavaScript:
- Async/await
Async/await is a new way to write asynchronous code in JavaScript. It allows developers to write code that is easier to read and understand, and it simplifies the process of handling asynchronous operations. Async/await is based on promises and provides a more intuitive syntax for working with promises.
async function fetchData() {
const response = await fetch('https://example.com/data')
const data = await response.json()
return data
}
fetchData().then((data) => {
console.log(data)
})
- Arrow functions
Arrow functions are a shorthand way to write functions in JavaScript. They have a concise syntax and can help make your code more readable. Arrow functions also have lexical scoping, which means they inherit the this keyword from the surrounding context.
const add = (a, b) => a + b
const result = add(2, 3)
console.log(result) // Output: 5
- Template literals
Template literals are a way to write strings in JavaScript that allows you to include expressions inside the string. They have a more readable syntax than traditional strings and can help make your code more concise.
const name = 'Ivan'
const age = 30
const message = `My name is ${name} and I am ${age} years old.`
console.log(message) // Output: 'My name is Ivan and I am 30 years old.'
- Spread operator
The spread operator allows you to expand an iterable, such as an array or a string, into individual elements. It can be used in many different ways and can help make your code more flexible and concise.
const arr1 = [1, 2, 3]
const arr2 = [4, 5, 6]
const mergedArr = [...arr1, ...arr2]
console.log(mergedArr) // Output: [1, 2, 3, 4, 5, 6]
Modern JavaScript has many cool features that can help developers write better, more efficient, and more maintainable code. These features can help reduce the amount of boilerplate code needed, improve the readability of code, and simplify complex operations. By leveraging these features, developers can create more robust and scalable applications that are easier to maintain and evolve over time.